SQL DB tool
By using the DB tool, you can connect your database and provide the model with valuable context, improving the quality of interactions. Learn more about the tool concept here
Here’s how you can do it:
Database support and configuration
Supported DB's
Ensure that your database is one of the following supported types:
- PostgreSQL (dialect:
postgresql
)- MySQL and MariaDB (dialect:
mysql
)- Oracle (dialect:
oracle
)- Microsoft SQL Server (dialect:
mssql
)- BigQuery
Password limitations
Please note that passwords should not contain the characters
@
and:
. If your password includes these characters, you will need to modify them in your connection string as follows:
- Replace
@
with%40
. For example:dialect://admin:My%[email protected]/dbtest
- Replace
:
with%3A
. For example:dialect://admin:My%[email protected]/dbtest
Database Query Limit Notice
To maintain optimal performance, each query is currently capped at a limit of 100 entries.
Postgres table schema
Please ensure that all column names are in lowercase with no capital letters.
Using the UI
Step 1: Add the DB tool
Click on the "+ Tool" button, and then SQL DB tool" start setting up the database connection.
Step 2: Configure the tool
- Assign a meaningful name to the tool. This name will help the model understand the context in which the data will be used.
- Add a description: Provide a thorough description of the database and its use. This information assists the model in contextualizing the data and influences the prompts generated by the system.
- Database Selection and Connection: Identify and connect to your chosen database by providing the requisite details:
- For BigQuery:
- Specify your
BigQuery project
. - Input the
BigQuery dataset
you intend to query. - Input your
GCP service account
information for credential validation (in a JSON format).
- Specify your
- For Other Database Types:
- Construct your Database URL in the format:
dialect://username:password@host:port/database
. Ensure you replace the placeholders with actual values specific to your database credentials and connection information.
- Construct your Database URL in the format:
- For BigQuery:
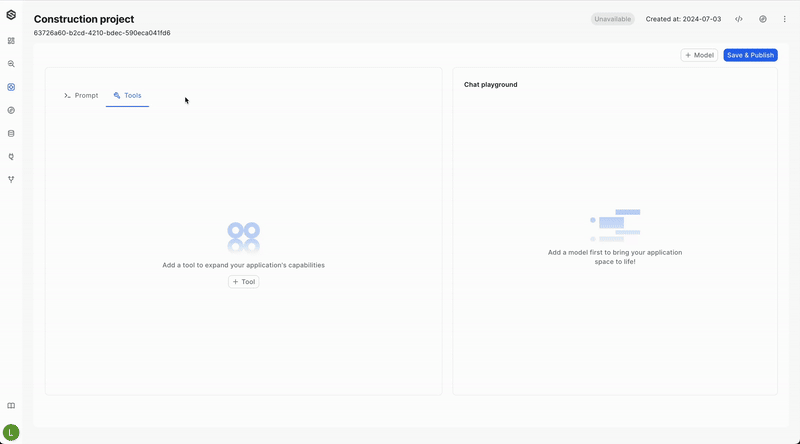
- Select specific tables for your tool: To enhance application performance and control access to specific tables in your database, you can configure your tool to access only selected tables. You have the flexibility to choose which tables your tool can access, grant access to all tables if needed, and enable automatic inclusion of any new tables added to the database.
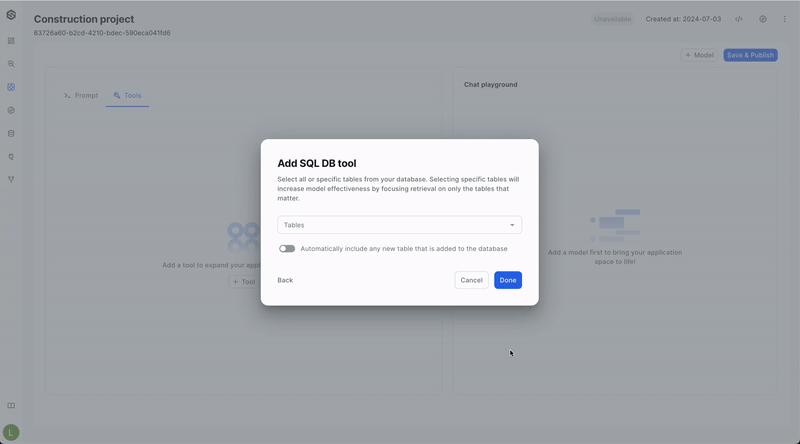
Using the SDK
To create a DB tool using the SDK, use the code snippet below as a guide and include the following details:
-
Assign a meaningful name to your tool. The chosen name will aid the model in recognizing the context for which the data is intended.
-
Provide a comprehensive description of the tool. Elaborate on the database’s purpose and its operational context. This description helps the model to contextualize the data, thereby enhancing the relevance and accuracy of the system-generated prompts
-
Connection configuration:
- For BQ db type: specify your
project_id
,dataset_id
andservice account
in a JSON format - For Other Database Types: Specify the connection string in the given format:
dialect://username:password@host:port/database
. Make sure to substitute the placeholders with your actual database credentials and details.
- For BQ db type: specify your
-
Select specific tables for your tool: To enhance application performance and control access to specific tables in your database, you can configure your tool to access only selected tables. You have the flexibility to choose which tables your tool can access, grant access to all tables if needed, and enable automatic inclusion of any new tables added to the database.
Create BQ DB tool example:
from superwise_api.models.tool.tool import ToolDef, ToolConfigBigQuery, ToolType, ToolConfigSQLMetadata
from superwise_api.models.application.application import AdvancedAgentConfig
bigquery_tool = ToolDef(
name="My tool name",
description="Describe this tool for the LLM",
config=ToolConfigBigQuery(
type=ToolType.SQL_DATABASE_BIGQUERY,
project_id="project_id",
dataset_id="dataset_id",
config_metadata=ToolConfigSQLMetadata(include_tables=["Tables to include"]), #Optional
service_account={SERVICE_ACCOUNT_JSON},
)
)
updated_app = sw.application.put(str(app.id),
llm_model=model,
prompt=None,
additional_config=AdvancedAgentConfig(tools=[bigquery_tool]),
name="My application name"
)
Create all other DB types tool example code:
from superwise_api.models.tool.tool import ToolDef, ToolConfigSQLDatabasePostgres, ToolConfigSQLMetadata, ToolType
from superwise_api.models.application.application import AdvancedAgentConfig
postgres_tool = ToolDef(
name="My tool name",
description="Describe this tool for the LLM",
config=ToolConfigSQLDatabasePostgres(
type=ToolType.SQL_DATABASE_POSTGRES,
config_metadata=ToolConfigSQLMetadata(exclude_tables=["Tables to exclude"]), #Optional
connection_string="[connection_string]",
)
)
updated_app = sw.application.put(
str(app.id),
llm_model=model,
prompt=None,
additional_config=AdvancedAgentConfig(tools=[postgres_tool]),
name="My application name"
)
ToolConfigSQLMetadata object
To select specific tables, you can use either the
include_tables
list or theexclude_tables
list:
include_tables
: Specify the tables you want to include. Note that any new tables added to the database will not be automatically included in this list.exclude_tables
: Specify the tables you want to exclude. This allows new tables added to the database to be automatically included in your tool's table list.- If you want your tool to access all tables, including any new tables added to the database, simply omit the
config_metadata
field when creating the tool. This configuration will be applied automatically.
Test connection
SWE offers you the option to check the connection to your resources in any given time by using the following API call
Example: Test connection to BQ
POST app.superwise.ai/v1/applications/test-tool-connection
{
"type": "BigQuery",
"project_id": "project_id",
"dataset_id": "dataset_id",
"service_account": {
"type": "service_account",
"project_id": "",
"private_key_id": "",
"private_key":"",
"client_email": "",
"client_id": "",
"auth_uri": "",
"token_uri": "",
"auth_provider_x509_cert_url": "",
"client_x509_cert_url":"",
"universe_domain":""
}
Updated 3 months ago